Sensor Hub¶
Sensor Hub is a sensor management component that can realize hardware abstraction, device management and data distribution for sensor devices. When developing applications based on Sensor Hub, users do not have to deal with complex sensor implementations, but only need to make simple selections for sensor operation, acquisition interval, range, etc. and then register callback functions to the event messages of your interests. By doing so, users can receive notifications when sensor states are switched or when data is collected.
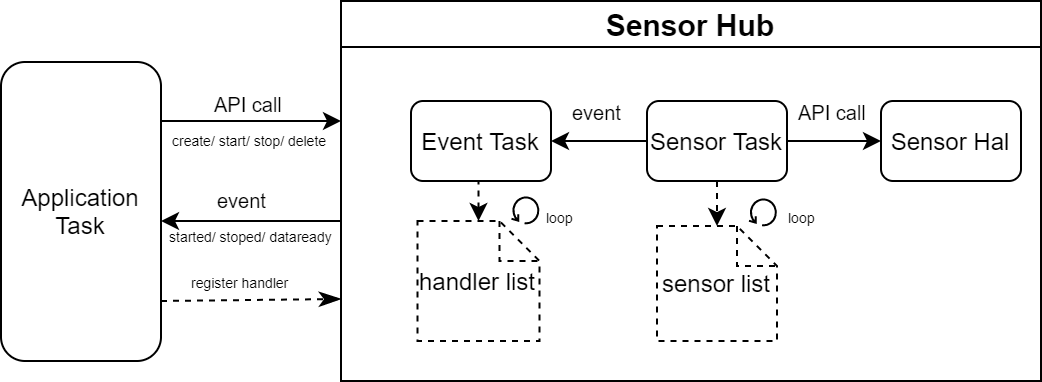
Sensor Hub Programming Model¶
The Sensor Hub provides hardware abstraction for common sensor categories, based on which users can switch sensor models without modifying the upper layer of the application. And it allows adding new sensors to the Sensor Hub by implementing their corresponding sensor interface at hardware abstraction layer. This component can be used as a basic component for sensor applications in various intelligent scenarios such as environment monitoring, motion detection, health management and etc. as it simplifies operation and improves operating efficiency by centralized management of sensors.
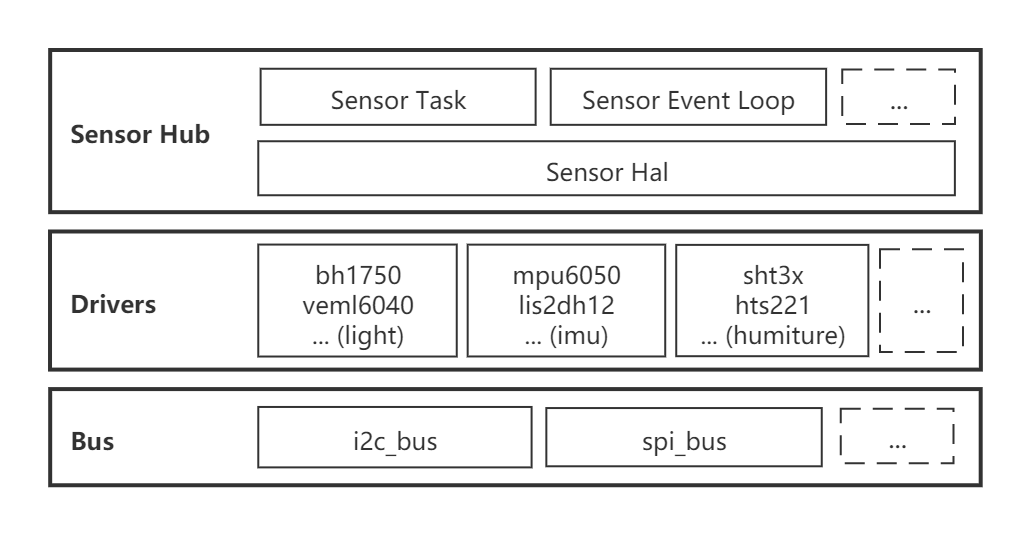
Sensor Hub Driver¶
Instructions¶
Create a sensor instance: use
iot_sensor_create()
to create a sensor instance. The related parameters include the sensor ID defined insensor_id_t
, configuration options for the sensor and its handler pointer. The sensor ID is used to find and load the corresponding driver, and each ID can only be used for one sensor instance. In configuration options, bus is used to specify the bus location on which the sensor is mounted; mode is used to specify the operating mode of the sensor; min_delay is used to specify the acquisition interval of the sensor, while other items inside are all non-required options. After the instance is created, the sensor handler is obtained;Register callback functions for sensor events: when a sensor event occurs, the callback functions will be called in sequence. There are two ways to register a callback function, and the instance handler of the event callback function will be returned after the registration succeed:
Use
iot_sensor_handler_register()
to register a callback function with the sensor handlerUse
iot_sensor_handler_register_with_type()
to register a callback function with the sensor type
Start a sensor: use
iot_sensor_start()
to start a specific sensor. After started, it will trigger aSENSOR_STARTED
event, then it will collect the sensor data continuously with a set of period and triggerSENSOR_XXXX_DATA_READY
event. The event callback function can obtain the specific data of each event via theevent_data
parameter;Stop a sensor: use
iot_sensor_stop()
to stop a specified sensor temporarily. After stopped, the sensor will send out aSENSOR_STOPED
event and then stop the data collecting work. If the driver of this sensor supports power management, the sensor will be set to sleep mode in this stage;Unregister callback functions for sensor events: the user program can unregister an event at any time using the instance handler of this event callback function, and this callback function will not be called again when this event occurs afterwards. There are also two ways to do so:
Use
iot_sensor_handler_unregister()
to unregister the callback function with the sensor handlerUse
iot_sensor_handler_unregister_with_type()
to unregister the callback function with the sensor type
Delete sensors: use
iot_sensor_delete()
to delete the corresponding sensor to release the allocated memory and other resources.
Examples¶
Sensor control LED example: sensors/sensor_control_led.
Sensor hub monitor example: sensors/sensor_hub_monitor.
API Reference¶
Header File¶
Structures¶
-
struct
sensor_data_t
¶ sensor data type
Public Members
-
int64_t
timestamp
¶ timestamp
-
uint8_t
sensor_id
¶ sensor id
-
int32_t
event_id
¶ reserved for future use
-
uint32_t
min_delay
¶ minimum delay between two events, unit: ms
-
axis3_t
acce
¶ Accelerometer. unit: G
-
axis3_t
gyro
¶ Gyroscope. unit: dps
-
axis3_t
mag
¶ Magnetometer. unit: Gauss
-
float
temperature
¶ Temperature. unit: dCelsius
-
float
humidity
¶ Relative humidity. unit: percentage
-
float
baro
¶ Pressure. unit: pascal (Pa)
-
float
light
¶ Light. unit: lux
-
rgbw_t
rgbw
¶ Color. unit: lux
-
uv_t
uv
¶ ultraviole unit: lux
-
float
proximity
¶ Distance. unit: centimeters
-
float
hr
¶ Heat rate. unit: HZ
-
float
tvoc
¶ TVOC. unit: permillage
-
float
noise
¶ Noise Loudness. unit: HZ
-
float
step
¶ Step sensor. unit: 1
-
float
force
¶ Force sensor. unit: mN
-
float
current
¶ Current sensor unit: mA
-
float
voltage
¶ Voltage sensor unit: mV
-
float
data
[4]¶ for general use
-
int64_t
-
struct
sensor_data_group_t
¶ sensor data group type
Public Members
-
uint8_t
number
¶ effective data number
-
sensor_data_t
sensor_data
[SENSOR_DATA_GROUP_MAX_NUM
]¶ data buffer
-
uint8_t
Macros¶
-
SENSOR_EVENT_ANY_ID
¶ register handler for any event id
Type Definitions¶
-
typedef void *
sensor_driver_handle_t
¶ hal level sensor driver handle
-
typedef void *
bus_handle_t
¶ i2c/spi bus handle
Enumerations¶
-
enum
sensor_type_t
¶ sensor type
Values:
-
NULL_ID
¶ NULL
-
HUMITURE_ID
¶ humidity or temperature sensor
-
IMU_ID
¶ gyro or acc sensor
-
LIGHT_SENSOR_ID
¶ light illumination or uv or color sensor
-
SENSOR_TYPE_MAX
¶ max sensor type
-
-
enum
sensor_command_t
¶ sensor operate command
Values:
-
COMMAND_SET_MODE
¶ set measure mdoe
-
COMMAND_SET_RANGE
¶ set measure range
-
COMMAND_SET_ODR
¶ set output rate
-
COMMAND_SET_POWER
¶ set power mode
-
COMMAND_SELF_TEST
¶ sensor self test
-
COMMAND_MAX
¶ max sensor command
-
-
enum
sensor_power_mode_t
¶ sensor power mode
Values:
-
POWER_MODE_WAKEUP
¶ wakeup from sleep
-
POWER_MODE_SLEEP
¶ set to sleep
-
POWER_MAX
¶ max sensor power mode
-
-
enum
sensor_mode_t
¶ sensor acquire mode
Values:
-
MODE_DEFAULT
¶ default work mode
-
MODE_POLLING
¶ polling acquire with a interval time
-
MODE_INTERRUPT
¶ interrupt mode, acquire data when interrupt comes
-
MODE_MAX
¶ max sensor mode
-
-
enum
sensor_range_t
¶ sensor acquire range
Values:
-
RANGE_DEFAULT
¶ default range
-
RANGE_MIN
¶ minimum range for high-speed or high-precision
-
RANGE_MEDIUM
¶ medium range for general use
-
RANGE_MAX
¶ maximum range for full scale
-
-
enum
sensor_event_id_t
¶ sensor general events
Values:
-
SENSOR_STARTED
¶ sensor started, data acquire will be started
-
SENSOR_STOPED
¶ sensor stoped, data acquire will be stoped
-
SENSOR_EVENT_COMMON_END
= 9¶ max common events id
-
-
enum
sensor_data_event_id_t
¶ sensor data ready events
Values:
-
SENSOR_ACCE_DATA_READY
= 10¶ Accelerometer data ready
-
SENSOR_GYRO_DATA_READY
¶ Gyroscope data ready
-
SENSOR_MAG_DATA_READY
¶ Magnetometer data ready
-
SENSOR_TEMP_DATA_READY
¶ Temperature data ready
-
SENSOR_HUMI_DATA_READY
¶ Relative humidity data ready
-
SENSOR_BARO_DATA_READY
¶ Pressure data ready
-
SENSOR_LIGHT_DATA_READY
¶ Light data ready
-
SENSOR_RGBW_DATA_READY
¶ Color data ready
-
SENSOR_UV_DATA_READY
¶ ultraviolet data ready
-
SENSOR_PROXI_DATA_READY
¶ Distance data ready
-
SENSOR_HR_DATA_READY
¶ Heat rate data ready
-
SENSOR_TVOC_DATA_READY
¶ TVOC data ready
-
SENSOR_NOISE_DATA_READY
¶ Noise Loudness data ready
-
SENSOR_STEP_DATA_READY
¶ Step data ready
-
SENSOR_FORCE_DATA_READY
¶ Force data ready
-
SENSOR_CURRENT_DATA_READY
¶ Current data ready
-
SENSOR_VOLTAGE_DATA_READY
¶ Voltage data ready
-
SENSOR_EVENT_ID_END
¶ max common events id
-
Header File¶
Functions¶
-
esp_err_t
iot_sensor_create
(sensor_id_t sensor_id, const sensor_config_t *config, sensor_handle_t *p_sensor_handle)¶ Create a sensor instance with specified sensor_id and desired configurations.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_id
: sensor’s id detailed in sensor_id_t.config
: sensor’s configurations detailed in sensor_config_tp_sensor_handle
: return sensor handle if succeed, NULL if failed.
-
esp_err_t
iot_sensor_start
(sensor_handle_t sensor_handle)¶ start sensor acquisition, post data ready events when data acquired. if start succeed, sensor will start to acquire data with desired mode and post events in min_delay(ms) intervals SENSOR_STARTED event will be posted.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_handle
: sensor handle for operation
-
esp_err_t
iot_sensor_stop
(sensor_handle_t sensor_handle)¶ stop sensor acquisition, and stop post data events. if stop succeed, SENSOR_STOPED event will be posted.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_handle
: sensor handle for operation
-
esp_err_t
iot_sensor_delete
(sensor_handle_t *p_sensor_handle)¶ delete and release the sensor resource.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
p_sensor_handle
: point to sensor handle, will set to NULL if delete suceed.
-
uint8_t
iot_sensor_scan
(bus_handle_t bus, sensor_info_t *buf[], uint8_t num)¶ Scan for valid sensors attached on bus.
- Return
uint8_t total number of valid sensors found on the bus
- Parameters
bus
: bus handlebuf
: Pointer to a buffer to save sensors’ information, if NULL no information will be saved.num
: Maximum number of sensor information to save, invalid if buf set to NULL, latter sensors will be discarded if num less-than the total number found on the bus.
-
esp_err_t
iot_sensor_handler_register
(sensor_handle_t sensor_handle, sensor_event_handler_t handler, sensor_event_handler_instance_t *context)¶ Register a event handler to a sensor’s event with sensor_handle.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_handle
: sensor handle for operationhandler
: the handler function which gets called when the sensor’s any event is dispatchedcontext
: An event handler instance object related to the registered event handler and data, can be NULL. This needs to be kept if the specific callback instance should be unregistered before deleting the whole event loop. Registering the same event handler multiple times is possible and yields distinct instance objects. The data can be the same for all registrations. If no unregistration is needed but the handler should be deleted when the event loop is deleted, instance can be NULL.
-
esp_err_t
iot_sensor_handler_unregister
(sensor_handle_t sensor_handle, sensor_event_handler_instance_t context)¶ Unregister a event handler from a sensor’s event.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_handle
: sensor handle for operationcontext
: the instance object of the registration to be unregistered
-
esp_err_t
iot_sensor_handler_register_with_type
(sensor_type_t sensor_type, int32_t event_id, sensor_event_handler_t handler, sensor_event_handler_instance_t *context)¶ Register a event handler with sensor_type instead of sensor_handle. the api only care about the event type, don’t care who post it.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_type
: sensor type decleared in sensor_type_t.event_id
: sensor event decleared in sensor_event_id_t and sensor_data_event_id_thandler
: the handler function which gets called when the event is dispatchedcontext
: An event handler instance object related to the registered event handler and data, can be NULL. This needs to be kept if the specific callback instance should be unregistered before deleting the whole event loop. Registering the same event handler multiple times is possible and yields distinct instance objects. The data can be the same for all registrations. If no unregistration is needed but the handler should be deleted when the event loop is deleted, instance can be NULL.
-
esp_err_t
iot_sensor_handler_unregister_with_type
(sensor_type_t sensor_type, int32_t event_id, sensor_event_handler_instance_t context)¶ Unregister a event handler from a event. the api only care about the event type, don’t care who post it.
- Return
esp_err_t
ESP_OK Success
ESP_FAIL Fail
- Parameters
sensor_type
: sensor type decleared in sensor_type_t.event_id
: sensor event decleared in sensor_event_id_t and sensor_data_event_id_tcontext
: the instance object of the registration to be unregistered
Structures¶
-
struct
sensor_info_t
¶ sensor information type
Public Members
-
const char *
name
¶ sensor name
-
const char *
desc
¶ sensor descriptive message
-
sensor_id_t
sensor_id
¶ sensor id
-
const uint8_t *
addrs
¶ sensor address list
-
const char *
-
struct
sensor_config_t
¶ sensor initialization parameter
Public Members
-
bus_handle_t
bus
¶ i2c/spi bus handle
-
sensor_mode_t
mode
¶ set acquire mode detiled in sensor_mode_t
-
sensor_range_t
range
¶ set measuring range
-
uint32_t
min_delay
¶ set minimum acquisition interval
-
int
intr_pin
¶ set interrupt pin
-
int
intr_type
¶ set interrupt type
-
bus_handle_t
Type Definitions¶
-
typedef void *
sensor_handle_t
¶ sensor handle
-
typedef void *
sensor_event_handler_instance_t
¶ sensor event handler handle
-
typedef const char *
sensor_event_base_t
¶ unique pointer to a subsystem that exposes events
-
typedef void (*
sensor_event_handler_t
)(void *event_handler_arg, sensor_event_base_t event_base, int32_t event_id, void *event_data)¶ function called when an event is posted to the queue
Enumerations¶
-
enum
sensor_id_t
¶ sensor id, used for iot_sensor_create
Values: