Button¶
The button component supports GPIO and ADC mode, and it allows the creation of two different kinds of the button at the same time. The following figure shows the hardware design of the button:
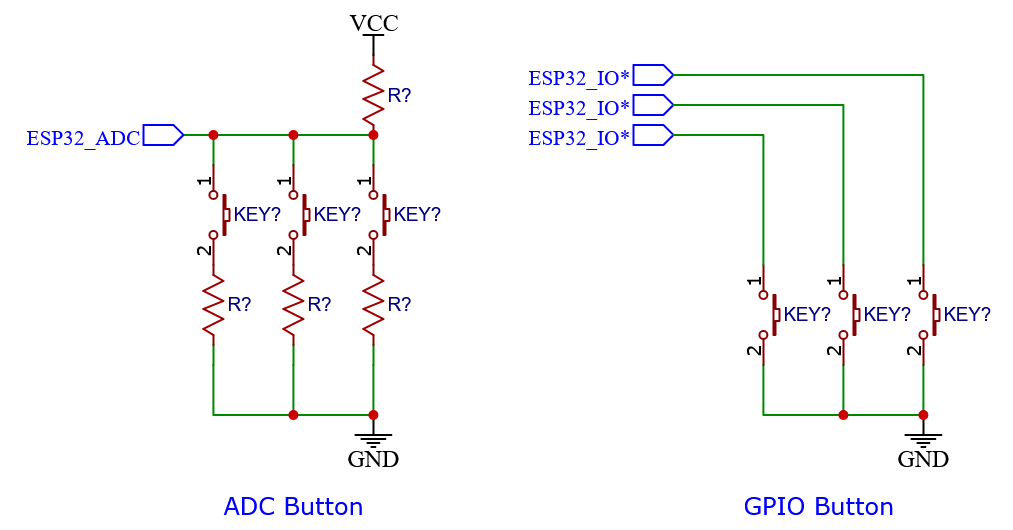
GPIO button: The advantage of the GPIO button is that each button occupies an independent IO and therefore does not affect each other, and has high stability however when the number of buttons increases, it may take too many IO resources.
ADC button: The advantage of using the ADC button is that one ADC channel can share multiple buttons and occupy fewer IO resources. The disadvantages include that you cannot press multiple buttons at the same time, and instability increases due to increase in the closing resistance of the button due to oxidation and other factors.
Note
The GPIO button needs to pay attention to the problem of pull-up and pull-down resistor inside the chip, which will be enabled by default. But there is no such resistor inside the IO that only supports input, external connection requires.
The voltage of the ADC button should not exceed the ADC range.
Button event¶
Triggering conditions for each button event are enlisted in the table below:
Event |
Trigger Conditions |
---|---|
BUTTON_PRESS_DOWN |
Button press down |
BUTTON_PRESS_UP |
Button release |
BUTTON_PRESS_REPEAT |
Button press down and release (>= 2-times) |
BUTTON_SINGLE_CLICK |
Button press down and release (single time) |
BUTTON_DOUBLE_CLICK |
Button press down and release (double times) |
BUTTON_LONG_PRESS_START |
Button press reaches the long-press threshold |
BUTTON_LONG_PRESS_HOLD |
Button press for long time |
BUTTON_PRESS_REPEAT_DONE |
Multiple press down and release |
Each button supports call-back and pooling mode.
Call-back: Each event of a button can register a call-back function for it, and the call-back function will be called when an event is generated. This method has high efficiency and real-time performance, and no events will be lost.
Polling: Periodically call
iot_button_get_event()
in the program to query the current event of the button. This method is easy to use and is suitable for occasions with simple tasks
Note
you can also combine the above two methods.
Attention
No blocking operations such as TaskDelay are allowed in the call-back function
Configuration¶
BUTTON_PERIOD_TIME_MS : scan cycle
BUTTON_DEBOUNCE_TICKS : debounce time
BUTTON_SHORT_PRESS_TIME_MS : short press down effective time
BUTTON_LONG_PRESS_TIME_MS : long press down effective time
ADC_BUTTON_MAX_CHANNEL : maximum number of channel for ADC
ADC_BUTTON_MAX_BUTTON_PER_CHANNEL : maximum number of ADC buttons per channel
ADC_BUTTON_SAMPLE_TIMES : ADC sample time
BUTTON_SERIAL_TIME_MS : call-back interval triggered by long press time
Demonstration¶
Create a button¶
// create gpio button
button_config_t gpio_btn_cfg = {
.type = BUTTON_TYPE_GPIO,
.long_press_ticks = CONFIG_BUTTON_LONG_PRESS_TIME_MS,
.short_press_ticks = CONFIG_BUTTON_SHORT_PRESS_TIME_MS,
.gpio_button_config = {
.gpio_num = 0,
.active_level = 0,
},
};
button_handle_t gpio_btn = iot_button_create(&gpio_btn_cfg);
if(NULL == gpio_btn) {
ESP_LOGE(TAG, "Button create failed");
}
// create adc button
button_config_t adc_btn_cfg = {
.type = BUTTON_TYPE_ADC,
.long_press_ticks = CONFIG_BUTTON_LONG_PRESS_TIME_MS,
.short_press_ticks = CONFIG_BUTTON_SHORT_PRESS_TIME_MS,
.adc_button_config = {
.adc_channel = 0,
.button_index = 0,
.min = 100,
.max = 400,
},
};
button_handle_t adc_btn = iot_button_create(&adc_btn_cfg);
if(NULL == adc_btn) {
ESP_LOGE(TAG, "Button create failed");
}
Note
please pass adc_handle and adc_channel , when there are other places in the project that use ADC1.
Register call-back¶
static void button_single_click_cb(void *arg,void *usr_data)
{
ESP_LOGI(TAG, "BUTTON_SINGLE_CLICK");
}
iot_button_register_cb(gpio_btn, BUTTON_SINGLE_CLICK, button_single_click_cb,NULL);
Find an event¶
button_event_t event;
event = iot_button_get_event(button_handle);
API Reference¶
Header File¶
Functions¶
-
button_handle_t
iot_button_create
(const button_config_t *config)¶ Create a button.
- Return
A handle to the created button, or NULL in case of error.
- Parameters
config
: pointer of button configuration, must corresponding the button type
-
esp_err_t
iot_button_delete
(button_handle_t btn_handle)¶ Delete a button.
- Return
ESP_OK Success
ESP_FAIL Failure
- Parameters
btn_handle
: A button handle to delete
-
esp_err_t
iot_button_register_cb
(button_handle_t btn_handle, button_event_t event, button_cb_t cb, void *usr_data)¶ Register the button event callback function.
- Return
ESP_OK on success
ESP_ERR_INVALID_ARG Arguments is invalid.
ESP_ERR_INVALID_STATE The Callback is already registered. No free Space for another Callback.
- Parameters
btn_handle
: A button handle to registerevent
: Button eventcb
: Callback function.usr_data
: user data
-
esp_err_t
iot_button_unregister_cb
(button_handle_t btn_handle, button_event_t event)¶ Unregister the button event callback function.
- Return
ESP_OK on success
ESP_ERR_INVALID_ARG Arguments is invalid.
ESP_ERR_INVALID_STATE The Callback is already registered. No free Space for another Callback.
- Parameters
btn_handle
: A button handle to unregisterevent
: Button event
-
size_t
iot_button_count_cb
(button_handle_t btn_handle)¶ how many Callbacks are still registered.
- Return
0 if no callbacks registered, or 1 .. (BUTTON_EVENT_MAX-1) for the number of Registered Buttons.
- Parameters
btn_handle
: A button handle to unregister
-
button_event_t
iot_button_get_event
(button_handle_t btn_handle)¶ Get button event.
- Return
Current button event. See button_event_t
- Parameters
btn_handle
: Button handle
-
uint8_t
iot_button_get_repeat
(button_handle_t btn_handle)¶ Get button repeat times.
- Return
button pressed times. For example, double-click return 2, triple-click return 3, etc.
- Parameters
btn_handle
: Button handle
-
uint16_t
iot_button_get_ticks_time
(button_handle_t btn_handle)¶ Get button ticks time.
- Return
Actual time from press down to up (ms).
- Parameters
btn_handle
: Button handle
-
uint16_t
iot_button_get_long_press_hold_cnt
(button_handle_t btn_handle)¶ Get button long press hold count.
- Return
Count of trigger cb(BUTTON_LONG_PRESS_HOLD)
- Parameters
btn_handle
: Button handle
Structures¶
-
struct
button_custom_config_t
¶ custom button configuration
Public Members
-
uint8_t
active_level
¶ active level when press down
-
esp_err_t (*
button_custom_init
)(void *param)¶ user defined button init
-
uint8_t (*
button_custom_get_key_value
)(void *param)¶ user defined button get key value
-
esp_err_t (*
button_custom_deinit
)(void *param)¶ user defined button deinit
-
void *
priv
¶ private data used for custom button, MUST be allocated dynamically and will be auto freed in iot_button_delete
-
uint8_t
-
struct
button_config_t
¶ Button configuration.
Public Members
-
button_type_t
type
¶ button type, The corresponding button configuration must be filled
-
uint16_t
long_press_time
¶ Trigger time(ms) for long press, if 0 default to BUTTON_LONG_PRESS_TIME_MS
-
uint16_t
short_press_time
¶ Trigger time(ms) for short press, if 0 default to BUTTON_SHORT_PRESS_TIME_MS
-
button_gpio_config_t
gpio_button_config
¶ gpio button configuration
-
button_adc_config_t
adc_button_config
¶ adc button configuration
-
button_custom_config_t
custom_button_config
¶ custom button configuration
-
union button_config_t::[anonymous] [anonymous]¶
button configuration
-
button_type_t
Type Definitions¶
-
typedef void (*
button_cb_t
)(void *button_handle, void *usr_data)¶
-
typedef void *
button_handle_t
¶